Author: | Carlos Santos |
Learning Line: | Programming |
Course: | PR1: Introduction to Programming |
Week: | 1 |
Competencies: | Students will be able to effectively define and use variables, programming flow control. |
BoKS: | 3bK2, The student understands the principles of data related software like Python, R, Scala and/or Java and knows how to write (basic) scripts. |
Learning Goals: | Variables and Data Types In-code Comments |
Introduction to Programming
Computer software programs is mainly a sequence of instructions, like following a cooking recipe.
This can be good and bad at the same time. The good is that gives you, the programmer, the possibility have a bit of freedom on how to create the master piece desert… but it also has is downside, you are not cooking the dish yourself but you have a servant that does all the work for you, and obeys your commands literary, sometimes too literally, leaving only you to blame for the mistakes in your instructions.
The basics
The first concept to understand about programming is that you declare a sequence of instructions, and they work like a queue. They will be performed based on the order they are provided.
Let’s take a look to the follow instruction sequence.
print('Hello World') print("I like cookies.") print('Bye!')
There are a few concepts that need to be understood.
- The order of instructions was the same as we declare them
- print is a function that outputs the content that you place between brackets ( ).
- We output strings in all print instructions. A string in programming represent text
Strings
In python strings can use single or double quotes. Some other programming languages use exclusively single or double quotes.
print('Single Quotes') print("double quotes")
Although, it is possible to use both notation, stick to one notation, it will make your code easier to read. Personally, I use double quotes, it is closer to the majority of other programming languages like C, C++, Java.
Simple Arithmetic
Looking back to the recipe analogy, you can look into strings, and numbers as base possible ingredients. In python, you can also output simple arithmetic operations. Numbers in general don’t have to be identified.
print(1) print(1+2) print(9-8) print(6*7) print(42/5) print(2**8) print( (45*3) / 4 - (5-3)**3 ) # make this complex arithmetic calculation
Basic arithmetic functions addition, subtraction, multiplication and division can be easy used (using the respective operators +, -, *, / ), and the respective mathematical rules apply (multiplication/division over addition/subtraction).
Exponent uses the operator **, and like in math you can use parenthesis do define the order of operations.
Note: you can add comments by adding # . Everything you type after a # is ignored. Comments are useful to help who is reading the code understand what it is being done. Use clear comments often.
Using Variables
Still sticking with the writing your own recipe. Variables can be viewed as measuring cups, with a couple of advantages:
- you can have as many as you want, and they are virtually limitless
- you don’t have to clean them, you can just throw them away
- they all have (different) names
milk = 180
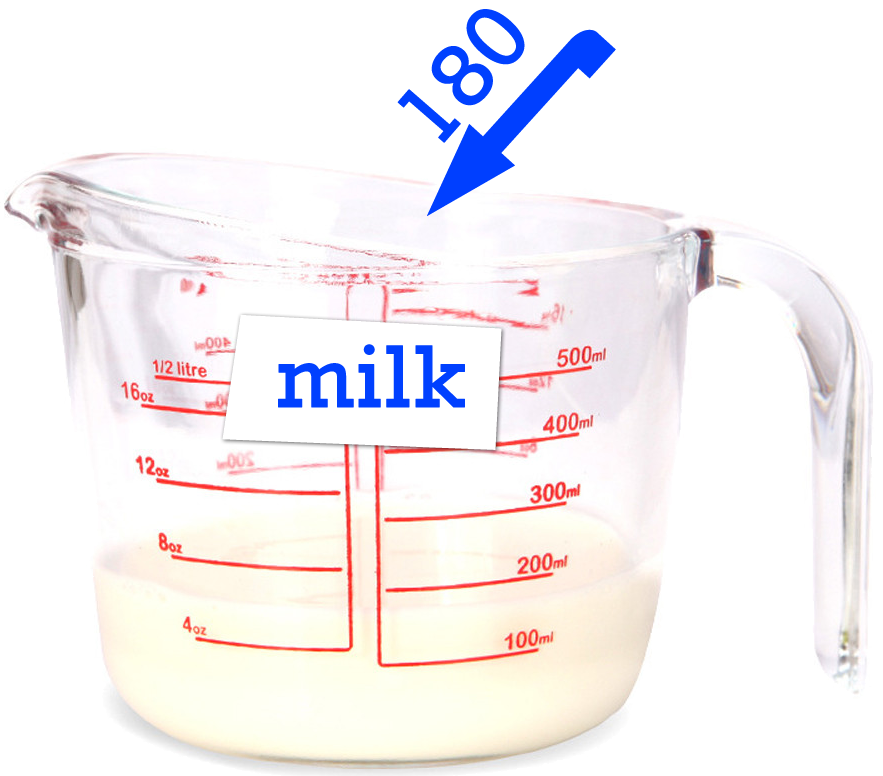
A similar concept exists in Math, in the sense they represent a value, in a mathematical function for example for us to represent lines. The main difference, is that in programming they hold a specific value, while in mathematical they normally represent a set of possible values.
Variables are a key concept, variables hold data, and they can be used and manipulated through instructions:
print(milk) # show the content of the variable milk milk = 20 # assign to milk the value of 20 print(milk + 30) # print the milk's value + 30 milk = milk + 10 # assign to milk the value of milk + 20
Note that code line 4, its not math, you could never write this using math, it would be absurd
In programming you can, because the = sign, is an attribution sign, not a comparison like in mathematics. In most programming languages, the comparison operator is == (double equal). In the case below, the result will be False
print(milk == milk + 10) # Compare (milk) with (milk + 10)
Data Types
Probably even without you realizing, we already addressed variable data types. So far, we talked about Strings, Number and Booleans. Those are the basic datatypes of Python, to be more exact:
- String’s – sequence of characters, or text
- Integer’s – which are numerical values without decimal points
- Float’s – which are numerical values with a floating comma
- Boolean’s – which may only be True or False
Data types are an important concept to understand, and these are only the fundamental ones, there are more, and with time you will be also creating your own to represent more complex concepts.
print( type("Hello") ) # show the type of a string print( type(1) ) # show the type of a non-decimal number print( type(1.3) ) # show the type of a decimal number print( type(True) ) # show the type of a boolean
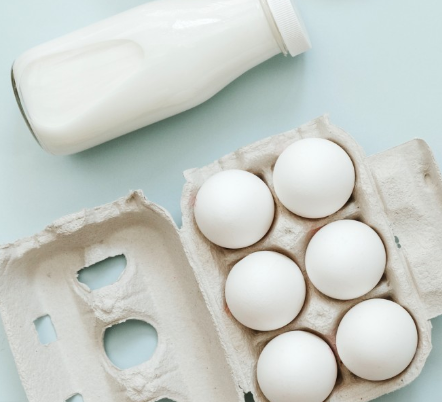
Variables also have a data type.
You can think of data-types as qualities required for your variables to have to hold your ingredients. You would not store milk in a egg carton.
# Pancake's recipe 4 persons milk = 0.18 print(type(milk)) sugar = 0.1 print(type(sugar)) flour = 0.18 print(type(flour)) sugar = 0.1 print(type(sugar)) eggs = 2 print(type(eggs)) salt = "a pintch" print(type(salt)) mixAll = True print(type(mixAll))
Converting data types
Like explained above your cooking servant is very literal. Everybody knows that for you to be able to add salt to eggs, it is implied that you break the egg before and you mean egg white and yoke (not the shell), well… your servant doesn’t.
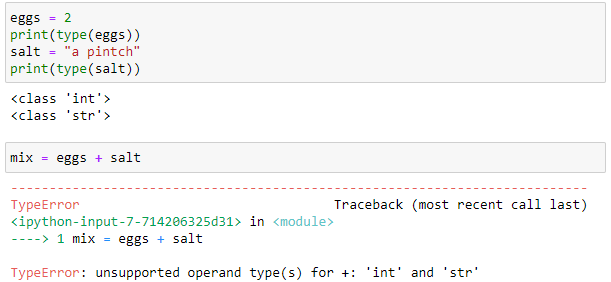
In short, eggs and salt variables are two different data types, and python needs to know how to deal with it. You have to explicitly say what needs to happen.

The str() function allows you to convert any data type into strings. Similar, functions can be found for integers, floats and boolean whenever possible.
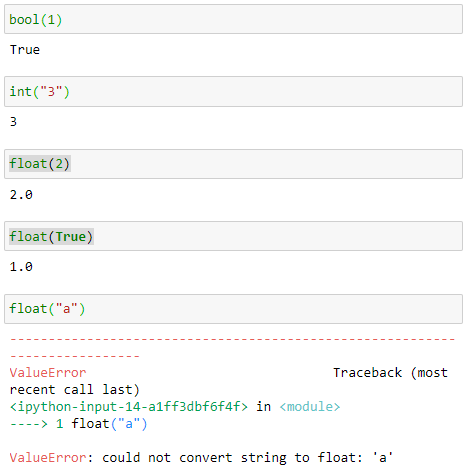
Input
User input is rather important, as such we will finish this preparation by introducing you to the input function, which allows you to request the user to type a value, which is can be stored in a variable (as a string).
userInput = input("type here> ") print (userInput) print(type(userInput))
To know more about variables and data types. Here is a couple of advised support videos.
Exercises
1. Write a python program to convert temperature from Fahrenheit to Celsius degree, for example 40°F = 4.44°C
2. Write a program to convert seconds into a number days, hours and minutes, for example 203 400 seconds = 2 days, 8 hours, 30 minutes
3. Write a program that accepts two integers from the user and then prints the sum, the difference, the product, the average.
4. Write a program to takes the user for a distance (in Kilometres) and the time was taken (as two numbers: hours, minutes), and display the speed, in meters per second and kilometres per hour.