Author: | Carlos Santos |
Learning Line: | Programming |
Course: | PR1: Introduction to Programming |
Week: | 3 |
Competencies: | Students will be able to effectively define and use variables, programming flow control. |
BoKS: | 3bK2, The student understands the principles of data related software like Python, R, Scala and/or Java and knows how to write (basic) scripts. |
Learning Goals: | Variables and Data Types In-code Comments |
Functions
A function is a block of code which only runs when it is called. You can pass parameters, into a function, and it can return data as a result.
You can see a function as a group of related instructions that performs a specific task. Functions help break our program into smaller chunks, it is the main way to support modular programming, and avoids repetition. In short makes the code reusable.
It as very similar concept in math:
can be written in python as:
def f(x, y): return x**2 + y f(2,3) 7 f(0,1) 1
The premises are similar, f is a function with two parameters x, and y. Parameters are special variables, normally required to calculate the result, of the function.
Functions are not required to have parameters or return values.
def printHello(): print("Hello function") printHello() Hello function
Parameter naming
The parameters names are important, since, you can use them to assure, you pass the correct information, and even reorder them is required.
def show3(n1, n2, n3): print("Show n1: " + str(n1)) print("Show n2: " + str(n2)) print("Show n3: " + str(n3)) show3(n3 = 3, n2 = 2, n1 = 1) Show n1: 1 Show n2: 2 Show n3: 3
Important: The instructions set of a functions all have the same level, meaning they all have the same trailing number of spaces/tabs. A function ends when the spacing returns to the previous level.
Parameter Default values
The parameters may have default values, it will assume the specified value if not specified. This is where the parameters names are also very important.
def g(a,b = 2, c= 1): return a**b-c g(3) 8 g(3,2,3) 6 g(50,0) 0 g(2,c=3) 1
Multiple return values
There is no limitations with regards to the number of return values, i.e., you can return more than one (not all programming languages allow this).
def getNeighbours(x): return x-1, x+1 getNeighbours(1) (0, 2) m, M = getNeighbours(3) print(m) 2 print(M) 4
Scopes
Whenever you define a variable within a function, its scope lies ONLY within the function. It is accessible from the point at which it is defined until the end of the function. Which means its value cannot be changed or even accessed from outside the function. Let’s take a simple example:
def functionScope(): x = 10 print("Value inside function:"+str(x)) x = 20 functionScope() print("Value outside function:"+str(x))
Note that in fact there are 2 variables declared, both are named x (line 2 and 5). They are in different scopes, one inside of the function (named functionScope), and the other in the base scope, known as global scope.
Scopes are important concepts to grasp, since, it might bring you a lot of trouble if they are not handled correctly. In Python they are illustrated by the spacing at the beginning of the instruction. This is called indentation.
Note that variables that are declared in a specific scope are available in sub-scopes.
a = 1 def functionThatUsesA(x): return x + a functionThatUsesA(3) 4
This type of programming is to be avoided, not only it is confusing, but, also it may create weird artifacts, and becomes difficult to read. The following code is valid, although difficult to read even for experienced programmers. So it is better to avoid it.
globalVar = 1 def functionScope1(): localVar1 = 2 localVar2 = 3 def functionScope2(): localVar1 = 4 localVar3 = 5 return globalVar + localVar1 + localVar2 + localVar3 return localVar1 + functionScope2() functionScope1()
Explanation:
- globalVar (orange) is a global variable reachable in all scopes
- localVar1 (blue) was defined inside functionScope1 and available both functionScope1 and functionScope2
- localVar2 (blue) was defined inside functionScope1 and available both functionScope1 and functionScope2
- localVar1 (pink) was defined inside functionScope2 and only inside functionScope2, and replacing the definition localVar1 (blue) inside functionScope2
- localVar3 (pink) was defined inside functionScope2 and only inside functionScope2
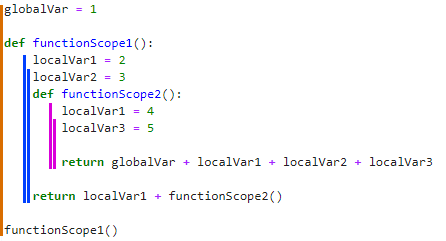
Function Documentation
Functions can (and should) be properly documented, this will help not only to understand the goal, of each function, but also clarify the role of each parameter of the function.
def increase(x, v = 1): """ This function increases the value of x, by the amount of v (default 1) """ return x + v increase(3) 4 help(increase) increase(x, v=1) This function increases the value of x, by the amount of v (default 1)
Documenting your functions is important, for supporting readability of your code.
Not all functions are required to be documented, but, it helps a lot to understand its purpose, and clarify any parameter, either for yourself or someone else.
Rule of thumb about documentation and code comment, answer this two questions:
– Will someone reuse this in the next two years?
– Will it take more time for him to understand what does the function do than me writing an explanation?
If for both question is a Clear No, then don’t bother. Otherwise, if it is a maybe or yes then document it.
Important to notice, that native functions from python are documented, meaning it has native documentation, by just typing help. In there, you can also see all the parameters.
help(print) Help on built-in function print in module builtins: print(...) print(value, ..., sep=' ', end='\n', file=sys.stdout, flush=False) Prints the values to a stream, or to sys.stdout by default. Optional keyword arguments: file: a file-like object (stream); defaults to the current sys.stdout. sep: string inserted between values, default a space. end: string appended after the last value, default a newline. flush: whether to forcibly flush the stream.
Explicit Types
You can explicit define parameter and return value types for functions. In some other programming languages this is mandatory, in python although it is possible it is uncommon among python programmers.
Personally, I only use them to increase even further readability, so myself or others will use this module often, or frequently and it may be obscure the parameter type.
def h(name: str, age : int) -> str: return name +" is "+str(age)+" years old"
Further Reading / Studying
https://www.programiz.com/python-programming/function
https://www.datacamp.com/community/tutorials/scope-of-variables-python
Exercises
1. Create a function that receives an integers and returns a boolean True, if it is odd and False if it is even.
tip: you can use the mod operator (%)
2. Create a function that calculates the Body Mass Index
3. Create a function that calculates the average of 5 float values.